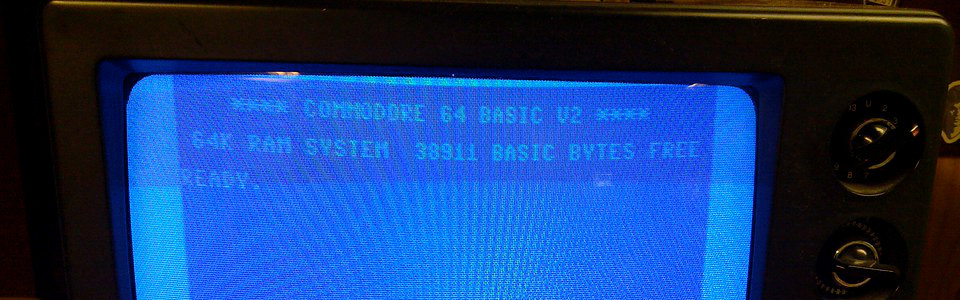
GOTO: Your Code's Teleportation Device
Ever wish you could jump around your code like a video game character? Say hello to GOTO
, your code's very own teleportation device! This command lets you instantly jump to a specific line number in your program, bypassing the normal sequential flow. While it might seem like a simple trick, it can be surprisingly powerful when used with care!
Syntax
GOTO <line number>
Where:
- <line number>
: The line number you want to jump to.
Applications
The GOTO
command can be used for:
- Creating loops: Combine
GOTO
with conditional statements (likeIF...THEN
) to create loops that repeat until a certain condition is met. - Error handling: Jump to specific error-handling routines when problems occur in your code.
- State machines: Create programs that transition between different "states" based on input or events.
Code Examples
1. Simple Jump:
10 PRINT "Start"
20 GOTO 50 :rem Jump to line 50
30 PRINT "This won't be printed"
40 END
50 PRINT "End" :rem Program continues here
This example shows how GOTO
skips over line 30 and jumps directly to line 50.
2. Conditional Loop:
10 INPUT "Enter a number (0 to quit): "; N
20 IF N=0 THEN END :rem Exit if the user enters 0
30 PRINT N*N :rem Print the square of the number
40 GOTO 10 :rem Go back to the input line
Here, GOTO
creates a loop that keeps asking for input until the user enters 0.
GOTO in the Wild: The Choose-Your-Own-Adventure Creator
Imagine you're writing an interactive story for the Commodore 64. You could use GOTO
to jump between different sections of the story based on the player's choices, creating a branching narrative where the reader controls the outcome.
While GOTO
can be a powerful tool, it's important to use it judiciously. Overuse of GOTO
can lead to "spaghetti code" – a tangled mess of jumps that's difficult to understand and maintain. So, if you find yourself using GOTO
excessively, consider alternative structures like FOR
loops, GOSUB
subroutines, or even rethinking your program's logic altogether.
With that said, don't be afraid to experiment with GOTO
and see how it can add flexibility and control to your Commodore 64 programming!
Important Note: Remember that in Commodore 64 BASIC, line numbers can range from 0 to 63999.