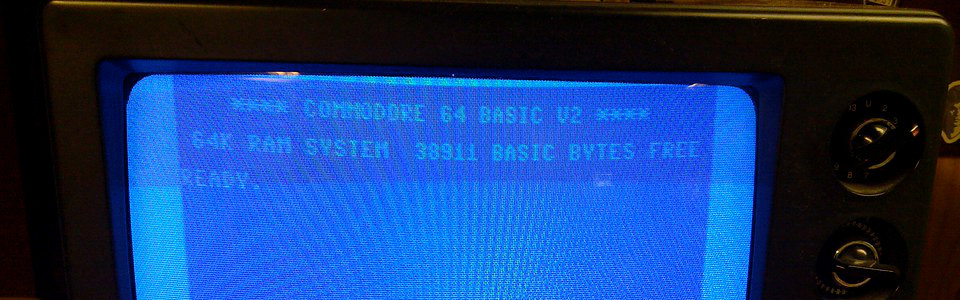
AND: The Logical Gatekeeper
Want to make sure multiple conditions are met before taking action? Need to filter out unwanted results? Say hello to the AND
operator! This logical champ lets you combine multiple tests, only giving you a thumbs-up if all conditions are true. It's like having a bouncer at your code's VIP party, making sure only the right guests get in.
Syntax
<condition 1> AND <condition 2> [AND <condition 3> ...]
Where:
- <condition>
: A logical expression that evaluates to either true (-1) or false (0). This can be a comparison (e.g., A < 10
), a variable holding a boolean value, or even the result of another AND
operation!
Applications
The AND
operator is your go-to tool when:
- You want to execute code only if several prerequisites are met.
- You need to create complex filters for data.
- You're building decision trees in your programs.
Code Examples
1. Simple Check:
10 A = 5
20 B = 12
30 IF A < 10 AND B > 10 THEN PRINT "Both conditions are true!"
Here, "Both conditions are true!" is only printed if A is less than 10 and B is greater than 10.
2. Multi-Level Filtering:
10 INPUT "Enter age: "; A
20 INPUT "Do you like pizza? (Y/N): "; P$
30 IF A >= 18 AND P$ = "Y" THEN PRINT "You're invited to the pizza party!"
This snippet checks both the age and pizza preference to decide whether someone gets a party invite.
3. Nested ANDs:
10 INPUT "Enter a number: "; N
20 IF N > 0 AND (N < 10 OR N > 20) THEN GOTO 40
30 END
40 PRINT "The number is positive and outside the range 10-20"
This example demonstrates how you can combine AND
with OR
for even more complex logic. Here, the number must be positive AND either less than 10 or greater than 20.
AND in the Wild: The Password Protector
Imagine you're coding a security system that requires a strong password. You could use AND
to check if the password meets multiple criteria:
- Is it at least 8 characters long?
- Does it contain both uppercase and lowercase letters?
- Does it include at least one number or special character?
Only if all conditions are met would the password be accepted.
Want to take your logical thinking to the next level?
AND
is your key to crafting intricate conditions and creating programs that make smarter decisions. It's like the duct tape of the programming world – versatile, reliable, and essential for sticking things together. So go ahead and experiment withAND
. Your code will be glad you did!